并发控制工具类-线程的3种创建方式
# 并发控制工具类
## Semaphore(5) 共享锁(只允许5个线程,并发)

```
package alex.smm.alipay.app.util;
import java.util.concurrent.Semaphore;
public class main {
static Semaphore sp = new Semaphore(5);
public static void main(String args[]){
for (int i=0; i<1000; i++){
new Thread(){
@Override
public void run() {
try {
sp.acquire(); //抢信号量、就是在加锁
Thread.sleep(2000L);
} catch (InterruptedException e) {
e.printStackTrace();
}
queryDB("localhost:3006");
sp.release(); //释放信号量,就是解锁
}
}.start();
}
}
public static void queryDB(String url){
System.out.println("query " + url);
}
}
```
## CountDownLatch 阻塞线程,等待其他任务全部执行完毕
```
package alex.smm.alipay.app.util;
import java.util.Random;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.Semaphore;
public class main {
static Semaphore sp = new Semaphore(5);
public static void main(String args[]){
try {
test01();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
/*
火箭起飞前,有很多检查需要做,每项检查需要的时间不同,
完成全部10项检查后,火箭才能点火
*/
public static void test01() throws InterruptedException {
CountDownLatch ctl = new CountDownLatch(10);
//任务在异步的执行
for (int i=0; i<10; i++){
int number = i;
new Thread(){
@Override
public void run() {
int randomInt = new Random().nextInt(10);
try {
Thread.sleep(randomInt * 1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(">>>>" + number);
//当任务执行完,将count-1
ctl.countDown();
}
}.start();
}
//通过await来阻塞住
System.out.println("主线程开始等待。。。");
ctl.await();
System.out.println("点火...");
}
}
```
## 线程的3种实现方式
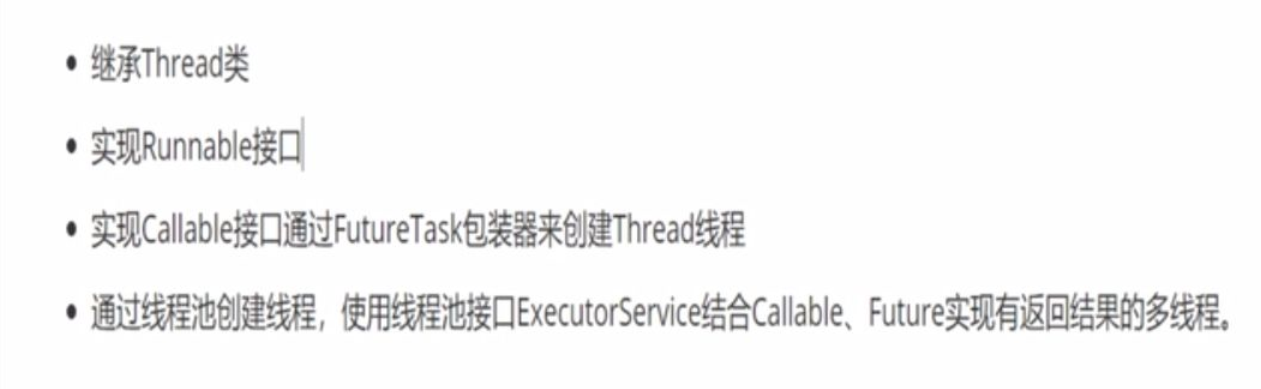
```
new Thread(){
@Override
public void run() {
System.out.println("dfasdfad");
}
}.start();
```
```
Runnable cmd = new Runnable() {
@Override
public void run() {
try {
Thread.sleep(10000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
};
cmd.run();
Thread th = new Thread(cmd);
th.start();
System.out.println("开启线程,实现异步,立即打印消息。。。");
```
```
package com.study.futuretask;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.FutureTask;
import java.util.concurrent.locks.LockSupport;
public class Demo3_CallableTest {
public static void main(String args[]) throws InterruptedException, ExecutionException {
//使用:用来包裹一个callab实例,得到的futureTask实例可以传入Thread()
CallableTask task = new CallableTask();
JamesFutureTask<String> future = new JamesFutureTask<>(task);
new Thread(future).start();
String result = future.get(); //get方法会阻塞
System.out.println(result);
//一个futureTask实例,只能使用一次
//同时说明,这个任务,从头到尾只会被一个线程执行
new Thread(future).start();
}
}
class CallableTask implements Callable<String>{
@Override
public String call() throws Exception {
System.out.println(">>>执行任务。。。");
//模拟耗时
LockSupport.parkNanos(1000 * 1000 *1000 * 5L);
return "success";
}
}
```