Server
.getScreenSize();
System.out.println( screenSize.getWidth() + " <长-宽> " + screenSize.getHeight());
```
## 获取鼠标坐标
```
Point point = java.awt.MouseInfo.getPointerInfo().getLocation();
x1=point.x;
y1=point.y;
```
## todo(备注 未完成任务)
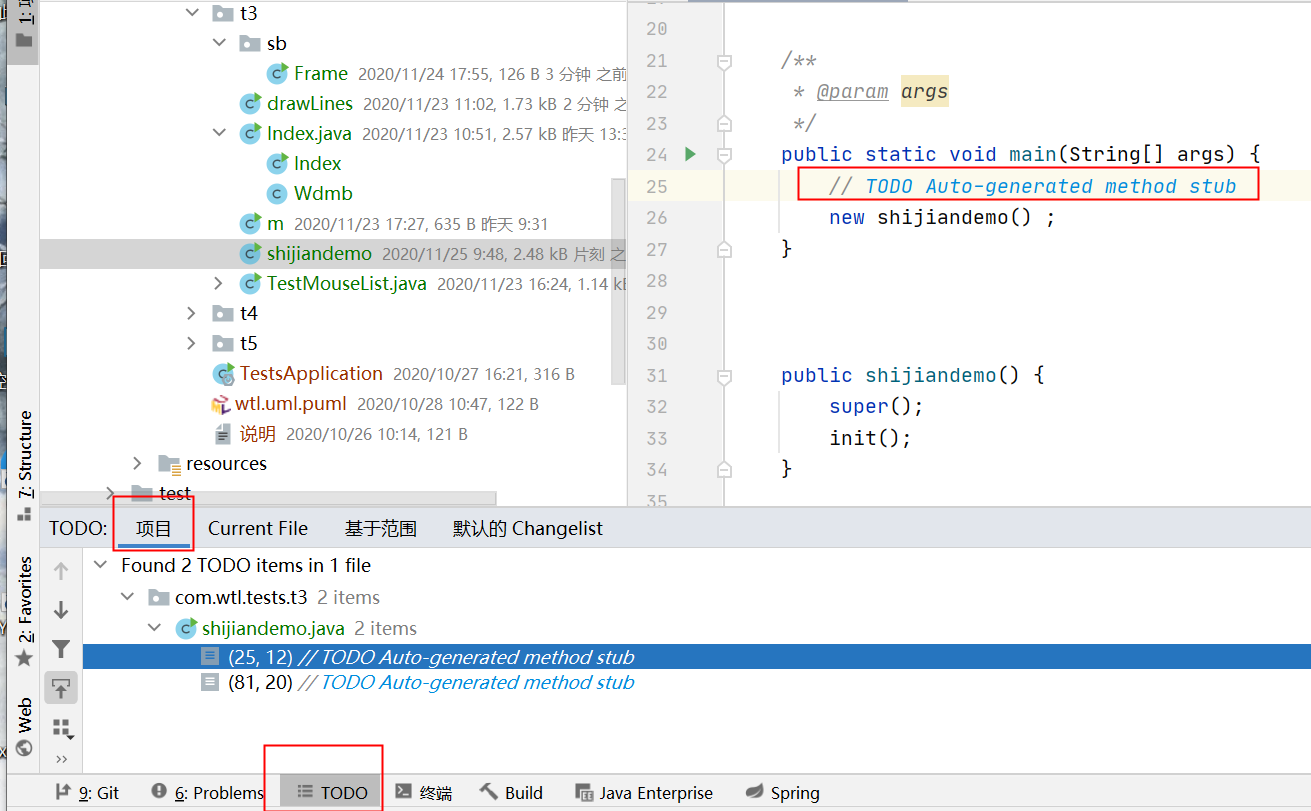
## 创建线程java la
```
new Thread(new Runnable() {
public void run() {
for(int i=0;i<20;i++) {
System.out.println("一边听歌");
}
}
}).start();
//jdk8 简化 lambda表达式
new Thread(()-> {
for(int i=0;i<20;i++) {
System.out.println("一边听歌");
}
}
).start();
```
## SimpleDateFormat
```
String date="";
SimpleDateFormat format=new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
date=format.format(new Date());
```
## java打开默认浏览器
```
Runtime.getRuntime().exec("cmd /c start http://wtl2.free.idcfengye.com/t1/get/all");
```
## length size 都是从1开始(从0开始循环<size length)
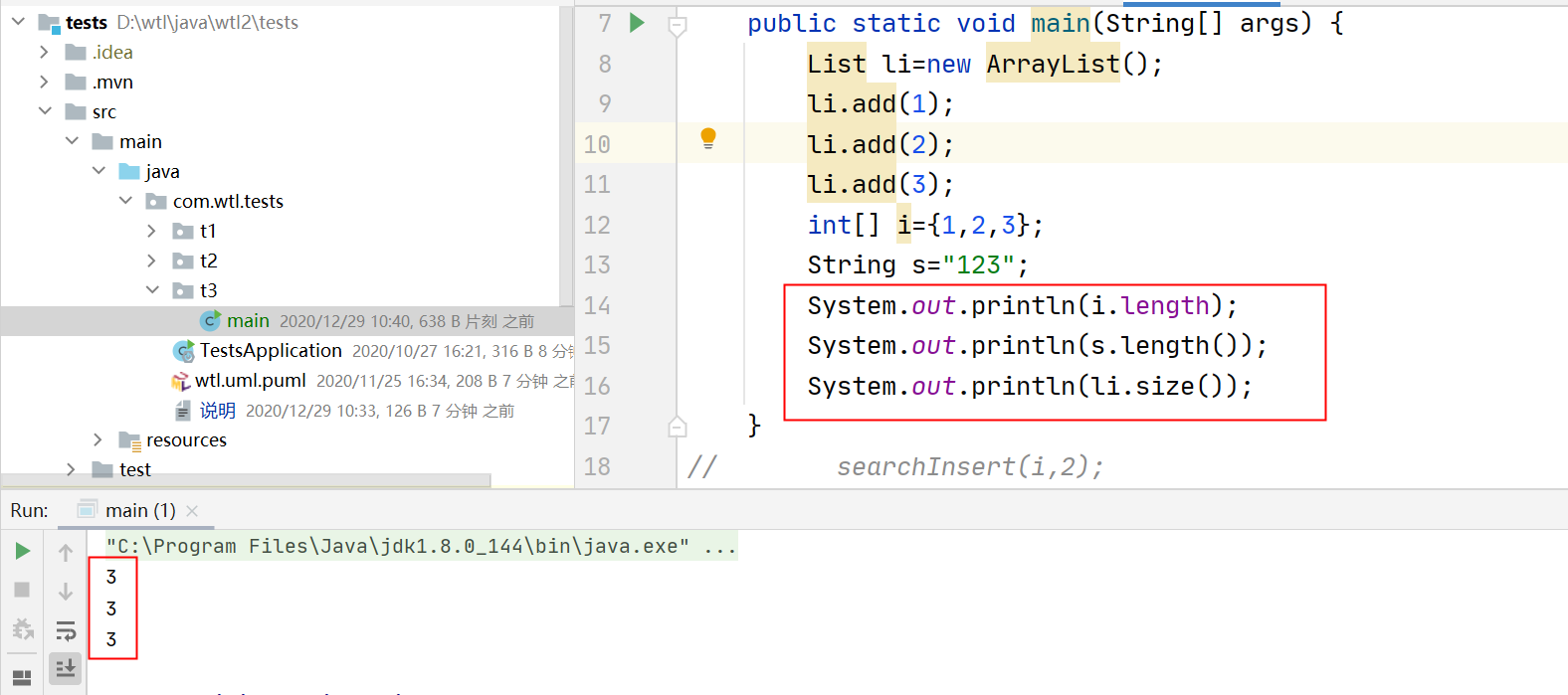
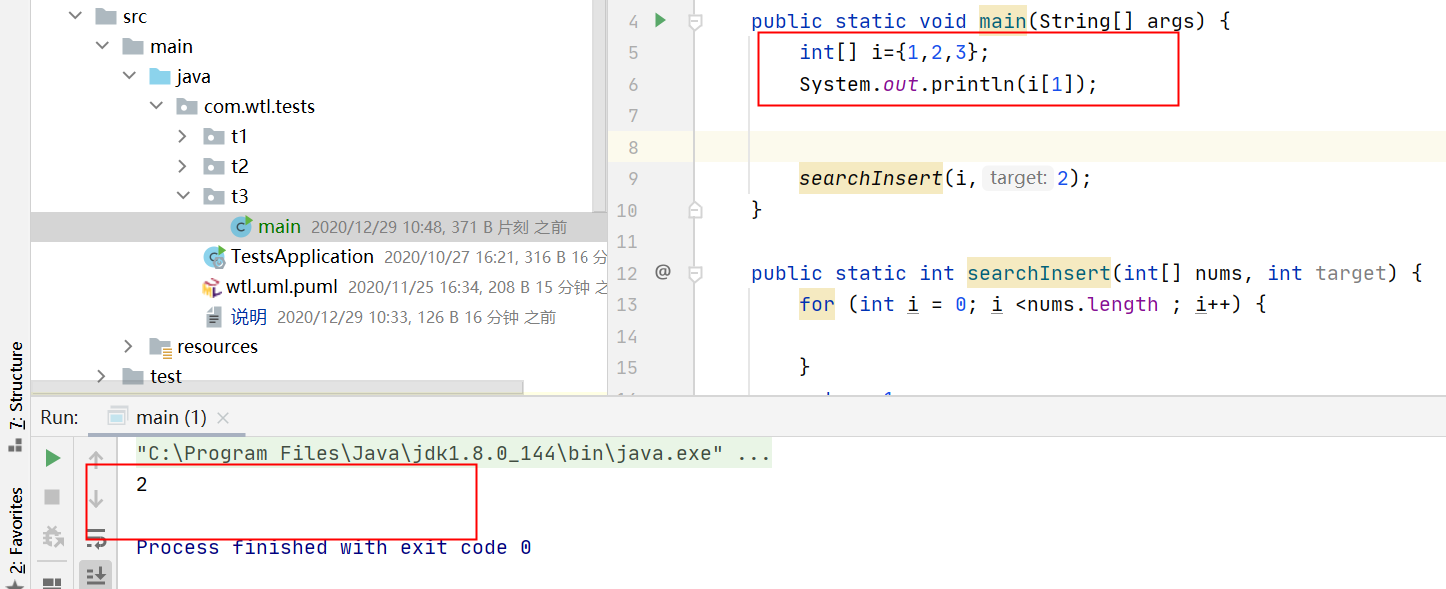
## json字符串转成对象 object转成json字符串
```
2、org.json
maven依赖如下
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20170516</version>
</dependency>
测试demo
import org.json.JSONObject;
public class OrgJsonDemo {
public static void main(String[] args) {
//创建测试object
User user = new User("李宁",24,"北京");
System.out.println(user);
//转成json字符串
String json = new JSONObject(user).toString();
System.out.println(json);
//json字符串转成对象
JSONObject jsonObject = new JSONObject(json);
String name = jsonObject.getInt("name");
Integer age = jsonObject.getInt("age");
String location = jsonObject.getInt("location");
User user1 = new User(name,age,location);
System.out.println(user1);
}
}
```
## hash算法 int小值-大值
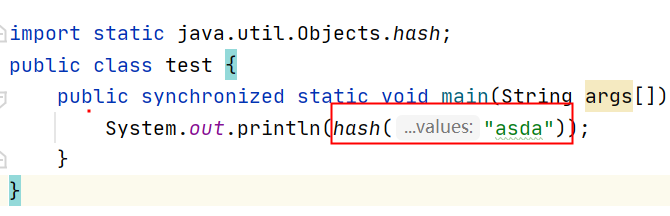
## 如何将字符串反转?
使用 StringBuilder 或者 stringBuffer 的 reverse() 方法。
示例代码:
```
// StringBuffer reverse
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append("abcdefg");
System.out.println(stringBuffer.reverse()); // gfedcba
// StringBuilder reverse
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append("abcdefg");
System.out.println(stringBuilder.reverse()); // gfedcba
```
## 如何实现数组和 List 之间的转换?
List转换成为数组:调用ArrayList的toArray方法。
数组转换成为List:调用Arrays的asList方法。
## 线程睡眠
LockSupport.parkNanos(1000 * 1000 * 1000L); //1s 线程等待1s
## 枚举使用
```
package com.cemoy.provider.membership.model.enums;
import lombok.Getter;
import org.apache.commons.collections4.CollectionUtils;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
@Getter
public enum DemoEnum {
NULL(null,""),
CEMOY(1, "cemoy"),
ANDRADA(2, "andrada");
private Integer code;
private String name;
DemoEnum(Integer code, String name) {
this.code = code;
this.name = name;
}
public static DemoEnum getEnum(int code) {
for (DemoEnum value : values()) {
if (Objects.equals(value.getCode(), code)) {
return value;
}
}
return NULL;
}
public static List<DemoEnum> filterByCodes(List<Integer> codes) {
if (CollectionUtils.isEmpty(codes)){
return Collections.emptyList();
}
List<DemoEnum> list = new ArrayList<>();
for (DemoEnum value : values()) {
if (codes.contains(value.getCode())) {
list.add(value);
}
}
return list;
}
}
```
## @Value @ConfigurationProperties(prefix = "shuyun.config")
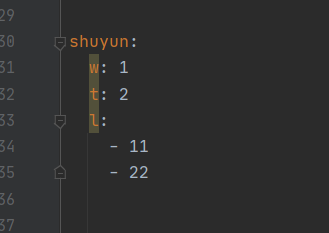
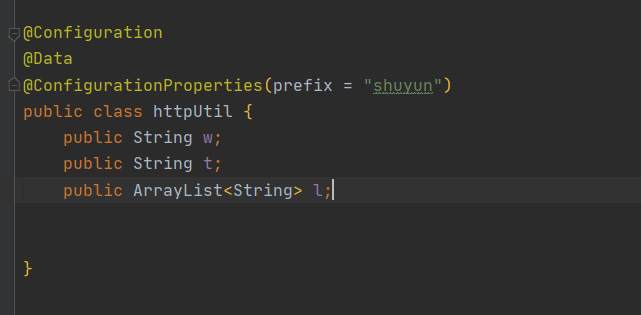
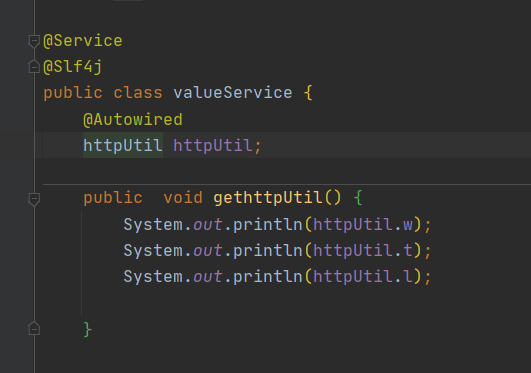
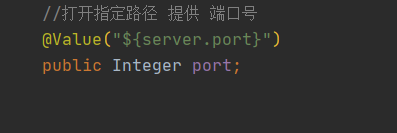
## java 8 stream
```
//双重排序
List<GoodsPriceItemTriVo> customerPriceItemList =null
return customerPriceItemList
.stream()
.sorted(Comparator.comparing(GoodsPriceItemTriVo::getErpCode, Comparator.reverseOrder())
.thenComparing(GoodsPriceItemTriVo::getType, Comparator.reverseOrder()))
.collect(Collectors.toList());
//分组 取最小
return customerPriceItemList.stream().filter(m -> m.getPrice() == null)
.collect(
Collectors.groupingBy(
GoodsPriceItemTriVo::getErpCode,
Collectors.minBy(Comparator.comparing(GoodsPriceItemTriVo::getPrice))
)
);
```
```
//排序转list
Map<String, Optional<GoodsPriceItemTriVo>> map=null;
return map.values()
.stream()
.filter(m -> m.isPresent())
.map(m -> m.get())
.sorted(Comparator.comparing(GoodsPriceItemTriVo::getErpCode).reversed())
.collect(Collectors.toList());
```
## redis @CreateCache
```
@CreateCache(expire = 60 * 60 * 24 * 30 * 3) //90天
public Cache<String, ShuYunCallBackQo> cache;
cache.get(key)
```
## instanceof 关键字的作用
```
//i必须是引用类型,不能是基本类型
Integer integer = new Integer(1);
System.out.println(integer instanceof Integer);//true
```
## Java 序列化中如果有些字段不想进行序列化
使用 transient 关键字修饰
。transient 只能修饰变量,不能修饰类和方法
## 有三个线程T1,T2,T3,如何保证顺序执行? join
```
final Thread t2 = new Thread(new Runnable() {
@Override
public void run() {
try {
// 引用t1线程,等待t1线程执行完
t1.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("t2");
}
})
```)